A microcontroller does not know what to do by itself. It’s your job to tell it what you want it to do. Microcontroller programming boils down to three steps:
- write program code on your computer
- compile the code with a compiler for the microcontroller you are using
- upload the compiled version of your program to your microcontroller
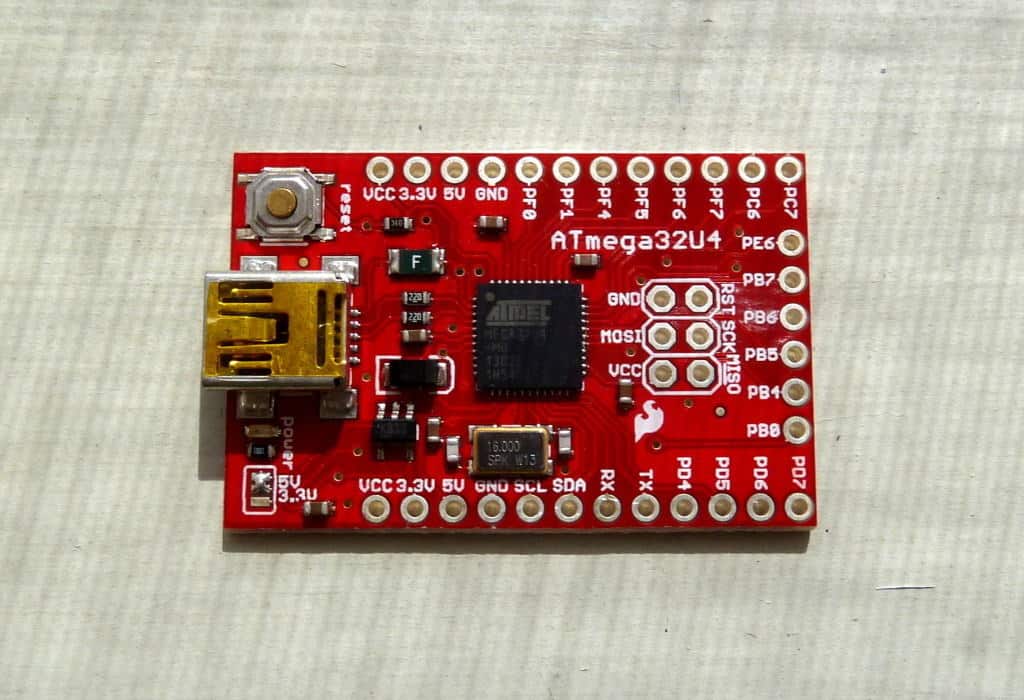
Write your program code
At the most basic level, a microcontroller understands only zeroes and ones (binary code). A program is a set of instructions written in a language the microcontroller can understand, telling it what to do with those zeroes and ones.
The first step is to write your program code. This is often done in C. But other languages are can work, depending on the microcontroller and its available compilers.
Key Building Blocks of Microcontroller Programming
Variables
Variables are like containers for storing data.
int age = 25; // Integer variable
float temperature = 26.5; // Floating-point variable
char initial = 'J'; // Character variable
bool isRunning = true; // Boolean variable (true/false)
Operators
Operators perform actions on variables and values.
int sum = 10 + 5; // Addition
int result = 10 % 3; // Modulo (gives the remainder: 1)
bool isEqual = (10 == 10); // Comparison (isEqual will be true)
Comments
Sometime it’s useful to have comments to let other programmers (or your future self) know what you are trying to do with the code. You can use single line comments or multiline comments.
// This is a single-line comment in C
/*
This is a multi-line
comment in C
*/
Control Flow
Conditional Statements:
Conditial statments are ways to do one thing if one statement is true (like a button being pressed) or another thing if not.
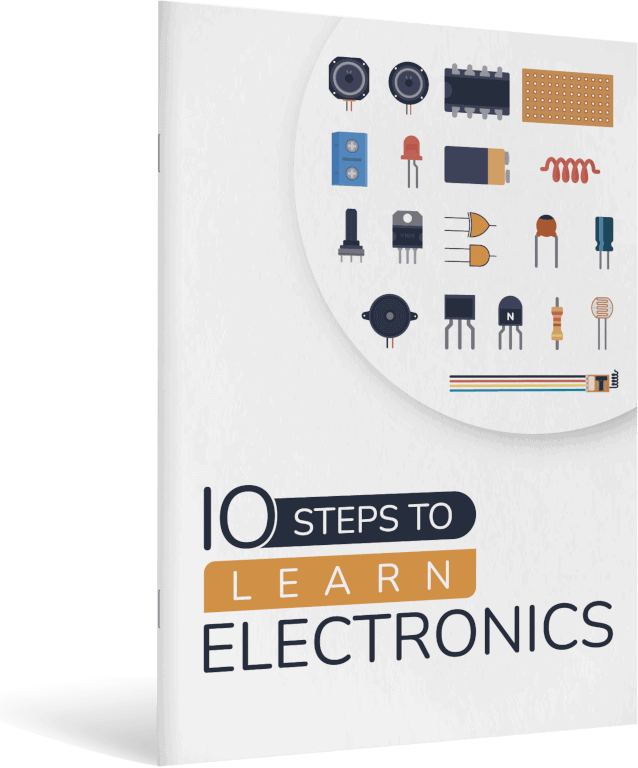
10 Simple Steps to Learn Electronics
Electronics is easy when you know what to focus on and what to ignore. Learn what "the basics" really is and how to learn it fast.
if (temperature > 30) {
// Code to execute if temperature is greater than 30
}
else if (temperature < 20) {
// Code to execute if temperature is less than 20
}
else {
// Code to execute if none of the above conditions are true
}
Loops:
Loops are ways to go through a series of numbers or other type of data. It can also be used to do something a certain number of times, like blinking an LED ten times.
for (int i = 0; i < 5; i++) {
// Code to repeat 5 times
}
while (isRunning) {
// Code to repeat as long as isRunning is true
}
Functions
You can create you own functions that do something specific. This is especially useful if you want to do the same thing several places in your code so that you don’t have to repeat the code so many times.
int calculateArea(int length, int width) {
int area = length * width;
return area;
}
Software For Writing Code
It doesn’t matter what software you use to write code. You can even use Notepad for this step. I like to use a really simple editor. But one that supports syntax highlighting makes the coding a bit easier. For Windows, my favorite is Notepad++
Arduino programming is a bit easier if you want to start really simple.
Compile your code for your microcontroller
Before you can upload your program to your microcontroller, you need to compile it. This means converting the code from human-readable code (words that make sense) to machine-readable code (1s and 0s).
Use a compiler that supports your microcontroller and compile your code into machine-code for your chip. A popular compiler for Atmel AVR microcontrollers is avr-gcc. Or if you’re using an Arduino, the compiler is included in the Arduino IDE.
After compilation, you will have one or more files containing machine code. Then you need to upload these files to your microcontroller. If you’re using the Arduino IDE, you just click on the Upload button.
Upload the compiled file(s) to you microcontroller
Usually, it’s one program file and a file for EEPROM and/or flash that you need to upload.
You need a physical connection from your computer to your microcontroller. Either you can use a dedicated programmer (such as the AVRISP for AVR microcontrollers), or if you have a USB programmable chip you can program it with a USB cable (my preferred method).
And you need a program for uploading the file(s). For AVR chips, you can use AVRDUDE.
For Arduino, you use a USB cable. The programmer is already on the board and uploading the compiled files happens automatically.
Next Step
With the main steps of microcontroller programming fresh in mind, it’s time to start building. If you are starting out, I would recommend starting with a microcontroller board. Arduino is the simplest, but there are many more available.
You can find lots of code examples for Arduino in our Arduino section. Start from the Arduino Blink LED example and build from there.
I’ve aslo written a very popular 5-part Microcontroller tutorial that takes you through the steps of building your own USB-programmable microcontroller board from scratch.
More Microcontrollers Tutorials
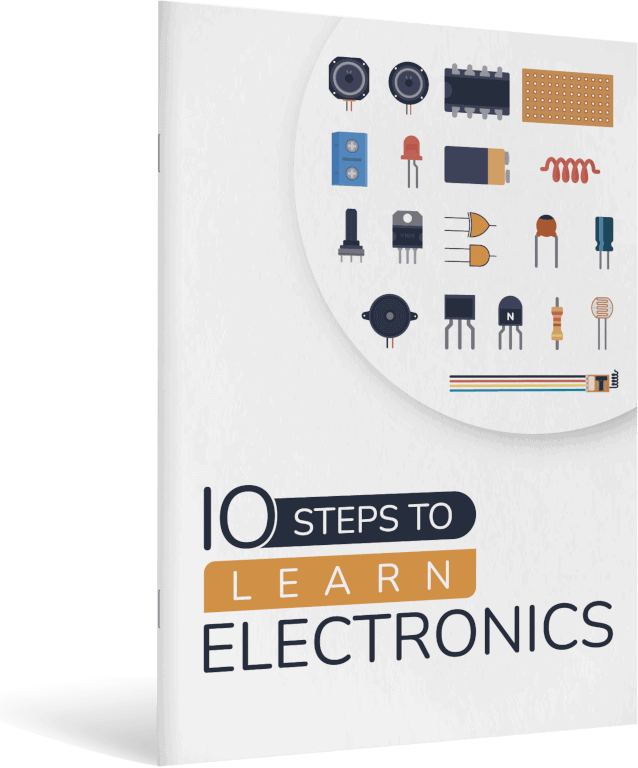
10 Simple Steps to Learn Electronics
Electronics is easy when you know what to focus on and what to ignore. Learn what "the basics" really is and how to learn it fast.